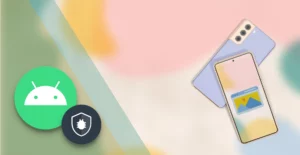
7 Minutes
ImageView in Android: Everything You Need to Know
Fix Bugs Faster! Log Collection Made Easy
Introduction
In Android, ImageView is a widget used to show an image, so it’s vital for every kind of app we build. In fact, it’s the most common widget that we’ll use as Android developers.
The image can be a profile image, graphic, or any other type of image, and it can be loaded from a res/drawable
folder or an external source. ImageView is pretty straightforward once you’ve got the hang of it, but there’s some stuff we need to know if we’re going to make the most of the widget.
In this article we’re going to delve right into it, looking at stuff like
- Loading external source images.
- How to customize images to our requirements.
- How to distinguish between ImageView and ImageButton (one of the most common painpoints for devs who are new to these concepts).
Table of Contents
What you’ll get from this article
You’ll get an excellent grounding in ImageView, which will provide the following benefits to your project:
- All the loading and scaling of images will be taken care of.
- You’ll have a fantastic tool to customize your image.
1. The basics of using ImageView in Android
There are two key types of image we will handle with ImageView. Here are the basics for each one:
- Static Image: For static images, you need to store the images in a
drawable
ormipmap
folder in your project, under theres
directory. You can also load device images in your app statically, either programmatically or by implementing a third-party library. - Dynamic Image: Dynamic images are loaded through a URL. To download an image from a URL, you can call on third-party libraries like Glide or Picasso, which are both very commonly used.
Loading an external source image
To load an external source image, you can use one of those third-party libraries we mentioned earlier (Picasso, Glide, etc), and there are some basic code strings which can really help us here.
To load an image from the res/drawable
folder, you need to write a single-line code in your layout file under the <ImageView></ImageView>
tag.
Here’s some code that can help us set an image from the res/drawable
folder in ImageView.
android:src="@drawable/img_profile_image"
img_profile_image
is the name of the image file, and it’s located in the res/drawable folder in your project.
Loading images from external resources
Now, here’s how to load images from external resources using third-party libraries:
Glide.with(context).load("IMAGE LINK").into(imageView)
// context = app context
// Image Link = where the image is stored
// imageview = ImageView object
To install Glide
in your project, you need to sync the library for the Gradle file.
repositories {
google()
mavenCentral()
}
dependencies {
implementation 'com.github.bumptech.glide:glide:4.16.0'
}
Picasso.get().load("Image Link").into(imageView)
// Image Link = where the image is stored
// imageview = ImageView object
How to present and animate images
To ensure the best possible UI, we need images that reflect our brand and enhance the user experience with their look, shape and feel.
Some app projects will simply require us to display basic images, but others will require us to enhance those images with specific colors, annotations or presentation styles, like slideshows.
Here are some essential concepts to bear in mind:
- Profile Image: Many apps display profile image as circles, which can look more professional than other shapes. If you want to follow this design style, you can use the
CircleImageView
library for rounder images. - Image Animation: Android provides a build-in animation API to show an animated image in ImageView. Various options are available to make the animation look more professional, including
fade-in
,fade-out
,zoom
,rotate
,slide,
etc. And here’s some code to get you on your way.
imageView.startAnimation(AnimationUtils.loadAnimation(context, R.anim.fade_in))
- Galleries or Sliding Images: To create a photo gallery in the app, we can use ImageView with
RecyclerView,
or create an image carousel usingViewPager
. - Placeholder Image: A placeholder is the image that displays before an intended image loads. Again, ImageView can assist here, if we use the following code:
Glide.with(context)
.load("Image link")
.placeholder(R.drawable.placeholder_image)
.into(imageView)
- Error Image: An error image is important for our communication with our users, giving them a clear signal that an intended image hasn’t loaded from its end point. Here’s how ImageView can help:
Glide.with(context)
.load("Image link")
.error(R.drawable.error_image)
.into(imageView)
- QR or Bar Code Display: A QR code can be scanned by a smartphone camera to perform a variety of functions, including opening a website URL or making a contactless payment. It can also enable users to scan for product details. To show a QR code you do not need anything extra, you can use an
ImageView
as long as you have the QR code in an image file. - Splash or Welcome Screen:
ImageView
can be deployed to show the app logo or welcome graphics in our app.
2. How to customize using ImageView in Android
In Android, ImageView
is a highly customizable and useful widget. There are many properties available to modify and display images, helping us get the right look whatever our needs might be. Here are the key properties:
- Layout Properties: This helps us set the
ImageView
position and size in the app, usinglayout_width
andlayout_height
. We can also uselayout_margin
to set the surrounding space.
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
- Content Properties: This helps us control the display of images in ImageView.
src
is used to set the path of the image in ImageView, whileadjustViewBounds
is used to set the aspect ratio of the image when resizing. Atint
is used to change the color of the image.
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/image" />
- Scale Type: This property helps us determine how the image will fit within
ImageView
.center
is used to center the image without scaling, whilecenterCrop
is used to crop the image and place it in the center of ImageView. If we want to fit the image within the ImageView and maintain the aspect ratio, it’scenterInside
. Or if we want to fit the image in ImageView and ignore the aspect ratio, it’sfitXY .
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/image"
android:scaleType="centerCrop" />
- Common Properties:
background
is used to set either the background image or the background color of ImageView, whilepadding
sets the internal space and specific edge spaces. Four distinct types of padding properties are available:paddingStart
,paddingEnd
,paddingTop
,paddingBottom
.visibility
properties help to control the visibility, whileid
is used to set the unique ImageView ID.
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/image"
android:scaleType="centerCrop"
android:visibility="gone"/>
- Runtime Properties: We can set the image from resource, drawable, or URI in ImageView at runtime by writing the code in a Java/Kotlin file.
setImageResource()
is used to set the image using a resource ID, whilesetImageBitmap()
properties helps to display the image using bitmap.setImageDrawable()
properties show images from the drawable folder of a project, andsetImageURI()
shows an image in ImageView using URI.
val imageView: ImageView = findViewById(R.id.imageView)
imageView.setImageResource(R.drawable.sample_image)
Now we’ve looked at ImageView’s specific functions and properties, we need to examine the differences between ImageView and ImageButton, as this can create problems if you’re not familiar with them.
Both ImageView and ImageButton are used to display an image, but they perform significantly different roles. The differences are as follows:
- ImageView is the best option when displaying a static image or icon.
- ImageButton is best when we need an image with text.
- ImageView does not provide clickable functionality by default, but ImageButton does.
- ImageView provides maximum customization for scaling an image, but ImageButton has limitations.
- ImageView takes less time to implement than ImageButton.
- ImageButton has built-in touch functionality, but ImageView requires custom styling.
Conclusion
ImageView is a very lightweight yet customizable Android widget that supports static and dynamic images. It provides various types of properties, helping the developer to fulfill their imaging requirements and create a professional app.
We can use ImageView in almost all places, like profile image, slideshow, animation, error handle, background and more.
Expect The Unexpected!
Debug Faster With Bugfender