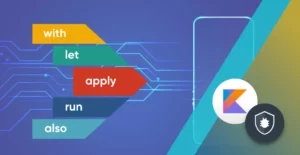
11 Minutes
Kotlin Apply and other Kotlin Scope Functions
Fix Bugs Faster! Log Collection Made Easy
Last week, we got a question from one of our users asking us how to use Kotlin Apply. Specifically, the reader wanted to know whether it was best to use the apply function in their Android application, or another of the many Kotlin scope functions.
So we got to thinking: Why not write an article about the whole topic of Kotlin scope functions? After all, they’re awesome: they let us write readable, concise code in Kotlin, and work with an object without the need for repeated references.
This not only cuts down boilerplate code, it provides a method to enhance the readability of our Kotlin apps and allow us to handle complex logic with minimal app maintenance. And if we want to build our own Kotlin DSL, then scope functions are absolutely crucial.
So today we’re going to cover all the main Kotlin scope functions (and yes, there’ll be a big section on the apply function) with practical examples and use cases. The blog is ideal for:
- Existing Kotlin developers who already know or have seen scope functions in their programming lives, but struggle to fully grasp them.
- Android developers who work with Kotlin and want to write more idiomatic, less verbose code in their next Android app, and explore object oriented programming.
- Java developers learning Kotlin and Kotlin multiplatform who want to drive their learning towards better practices in a new programming language.
One key thing you’ll learn is how to leverage scope functions in Android development, and why it is totally worth the effort for every Kotlin developer, whether they’re seasoned or new to the language.
Table of Contents
First, an introduction to scope functions
What are scope functions?
If you’re new, scope functions are provided by a Kotlin standard library to simplify working with an object in a scope, and make our code much more readable and easy to maintain.
They allow us to write code without writing the object reference every time, which can be a pain, and assist in realigning our code so that we can easily perform operations on an object, without polluting the base.
Types of scope functions
There are a total of five scope functions in Kotlin, each with their own distinct use cases and advantages. The higher-order functions we will cover today are let
, run
, with
, apply
and also
. Here is a really quick deep-dive on each one:
let
:
Let is used to run blocks of code on object, and then return the result or follow up with other operations in a chain (see example below), as well dealing with null safely. Here’s a code snippet to show you what we mean.
val user = User("John", "Doe")
user?.let {
Log.d("User", "First name: ${it.firstName}, Last name: ${it.lastName}")
}
run
:
Run is great because it combines the work of let and functions in tandem, facilitating both side effects while returning a value.
val fullName = user.run {
"$firstName $lastName"
}
Log.d("User", "Full name: $fullName")
with
:
This function lets us perform an operation on a object without actually extending it. Like run, this is a non extension function (i.e. it doesn’t extend another class or type), and it requires the object as its parameter.
with(user) {
Log.d("User", "First name: $firstName")
Log.d("User", "Last name: $lastName")
}
apply
:
Now, we’re back to what we discussed at the top. Apply is a constructor, which is used to set up an object and return the object itself.
val user = User().apply {
firstName = "John"
lastName = "Doe"
}
Log.d("User", "User created: $user")
also
:
This is the same as the apply function, but for side-effects, like logging or validation. It returns the object itself.
val user = User("John", "Doe").also {
Log.d("User", "User created: $it")
}
Comparing scope functions
The specific scope function to use depends on what you want to accomplish in your code. Here are some tips to choose the right one:
let:
We uselet
when we perform a certain operation with a non-null object or transform the result. This is great for chaining calls or working with nullable objects to prevent null pointer exceptions.user?. let { }
run:
This is great when we want to execute a block of code and get a receiver result from the last expression. Very useful for value object configuration and computation of your own.
val result = configuration.run { //do computations and return result. }
with:
With is particularly useful for chaining multiple function calls over an object without repeating a reference to the same thing. Useful if you are doing a number of things to an existing object.
with(configuration) { /* lots of operations */ }
apply:
Apply comes into its own when you need to initialize or configure the object and want the function to return itself. Great for constructor execution and object initialization.
val paint = Paint().apply { properties }
also:
We can usealso
to peform more operations on an object, like logging or debugging. Appropriate for operations returning the object itself, after performing the side effect.val file = File(path).also { Log.d("File", "File path: ${it.path}") }
Differences and similarities
Now let’s go deeper.
Each scope function carries its own explicit behavior, so let’s look at the specific use of each of them: in other words, where and when we can use these different scope functions in the Android app development pipeline. We will also explore the similarities (and differences) of the various functions along the way.
Context object:
let
&also
require the use ofit
as the context object in a lambda function.run
,with
andapply
require the use ofthis
as the context object in a lambda expression.
Return value:
let
&run
return the result of the lambda function.with
returns the lambda result.apply
andalso
return the object itself.
Usage pattern:
let
is used for null-checks and transformations.run
– is the computation and result return code.with
– is an operand that declares an already initialized object so you can perform a task with it.apply
is used to instantiate and configure objects.also
is used for side effects, like logging and additional operations that do not violate the main object.
Ok, that’s the basic primer done. Now let’s really dig into those weeds.
Advanced usage of scope functions
Now we’re really cooking! We’ve gone through all the definitions and distinctions, so let’s start looking at advanced use cases and seeing how the different scope functions can be used in tandem.
Nested scope functions
Nesting scope functions are great when performing complex object hierarchies. And with careful nesting of scope functions, you can maintain a clean, readable structure where operations related to each object are all inside the relevant block.
To show you what we mean, let’s look at a code snippet involving apply
and let
.
//Example of usng appy and let scope functions together
val user = User().apply {
firstName = "Sam"
lastName = "Doe"
}.let { user ->
// We can perform additional operations here
Log.d("User", "User is created: ${user.firstName} ${user.lastName}")
user
}
Chaining Scope functions
Now this bit is crucial. Let’s look at chaining functions together.
The most common way to chain scope functions is popping out of the last block, calling a new function and repeating that until we get what were expecting. It’s nice and simple, and is a really good way to avoid that dreaded boilerplate code.
As an example, here’s a code snippet showing how to chain apply
, also
and let
.
//Chaining example of the apply, also and let functions.
val userDisplayName = User().apply {
firstName = "Sam"
lastName = "Doe"
}.also {
Log.d("User", "User is created: ${it.firstName} ${it.lastName}")
}.let {
"${it.firstName} ${it.lastName}"
}
Log.d("User", "Display Name: $userDisplayName")
Now let’s say we want to set some properties for a UserProfile object, log its properties and then finally display a summary. How would we do this? Well, let’s get into the language.
//Example of UserProfile class that has user properties and showing the use of different scope functions.
data class UserProfile(
var firstName: String = "",
var lastName: String = "",
var age: Int = 0,
var email: String = ""
)
fun main() {
val userProfileSummary = UserProfile().apply {
firstName = "Sam"
lastName = "Doe"
age = 30
email = "[email protected]"
}.also { profile ->
Log.d("UserProfile", "Profile is created: $profile")
}.run {
"Name: $firstName $lastName, Age: $age, Email: $email"
}
Log.d("UserProfile", "The Profile Summary: $userProfileSummary")
}
Real-world use cases
The bit you’ve been waiting for, right? A goold old hand-ons tutorial.
In a real-world application of Android example, the code above will feature scope functions.
To build the use case, let’s start by creating a simple example where we have to create a user profile screen. These tasks often involve fetching user data, initializing views or displaying fetched data on screen.
To initialize views in a user profile screen and set them, use apply
.
class UserProfileActivity : AppCompatActivity() {
private lateinit var binding: ActivityUserProfileBinding
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityUserProfileBinding.inflate(layoutInflater)
setContentView(binding.root)
// Initialize views using apply
binding.apply {
userNameTextView.text = ""
userEmailTextView.text = ""
userProfileImageView.setImageResource(R.drawable.placeholder_profile)
}
// Fetch and display user data
fetchUserDataAndDisplay()
}
private fun fetchUserDataAndDisplay() {
val userRepository = UserRepository()
// Fetch user data using run
val user = userRepository.run {
getUserData()
}
// Display user data using let
user?.let {
binding.userNameTextView.text = "${it.firstName} ${it.lastName}"
binding.userEmailTextView.text = it.email
// Assuming you have an image loading library like Glide or Picasso
Glide.with(this).load(it.profileImageUrl).into(binding.userProfileImageView)
}
}
}
There we go! Not bad right? The code all flows smoothly (if we say so ourselves!) and it’s all nice and clear.
Best practices
Ok, we’re nearly at the finish line now. Let’s cap things off by exploring some best practices that we can use when working with scope functions in our Kotlin projects.
- Pick your scope function: Choose the appropriate scope function for your case. For example,
apply
is great for object initialisation,let
is good for null checks and to transform a value, andrun
is best on computations that return something. - Keep the code short: Never write large blocks of code into scope functions. The shorter your code, the more readable it will be, and the easier it will be to determine what the scope function does.
- Retain scope functions for a proper level of nesting: As nesting can be deeper down to the last level, you should not bury scope functions deep inside one another.
- Clarify context object naming: Use
let
oralso
where appropriate, When using thelet
keyword in a regular lambda, provide more meaningful names to the context object if necessary. - Use scope functions for null-safety:
let
is used to execute blocks of code on nullable objects and avoid null pointer exceptions. In the context of Android app development, we often have to work with value references, so this particular facet is really useful. - Use a scope functions chain: You can chain scope functions to make the code look more clean and readable. Each step or function in the chain should do one, and only one thing.
To sum up
Wow, we’ve covered a lot there right? We’ve discussed the key features of scope functions, we’ve introduced the basics behind scope functions and how they can make your Kotlin code more clean and concise, before detailing each scope function (let
, run
, with
, apply
, also
), its purposes and syntax and its use in real-life applications. We have also discussed the advanced usage of scope functions via nesting or chaining, to handle complex conditions more effectively.
Remember: scope functions are ultimately a way of writing clean, concise code in Kotlin. The better we utilize scope functions, the more bespoke (and less boilerplated) our code will look. Happy coding guys!
Expect The Unexpected!
Debug Faster With Bugfender