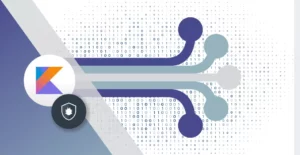
11 Minutes
Kotlin Switch Explained: Unlocking the Power of Kotlin when
Fix Bugs Faster! Log Collection Made Easy
The when
in Kotlin defines a conditional expression with more than one branch, that’s the statement you need to use when you want to write a Kotlin switch. In Java, we are using switch
case statements. In Java, we need to define a break
in each switch case, but in Kotlin we don’t need to. There are two ways you can use them in Kotlin. Either as a statement or as an expression.
Table of Contents
Before moving on to a Kotlin switch example, you need to be clear about switch expression and switch statement. Expression returns a value, but statement does not; it only performs an action. Let us create a Kotlin example to clearly explain the difference.
Difference between expression and statement in when
:
// expression example
fun main() {
val dayType = "Monday"
val result = when(dayType) {
"Sunday"->"Holiday"
"Saturday"-> "Half Day"
else-> "Full Working Day"
}
println("$dayType is $result ")
}
**Output: Monday is Full Working Day**
The value matched with the else block and returned.
// statement example
fun main() {
val dayType = "Saturday"
when(dayType) {
"Sunday"-> {
println("Holiday")
}
"Saturday"-> {
println("Half day")
}
else-> {
println("Full Working Days ")
}
}
}
**Output: Half day**
The value matches the else block and does not return. It only executes the print statement.
when
is used as single block:
To use common behavior for multiple cases, we can use commas (,)
to combine multiple conditions in a single block. Let us have a quick example to understand single blocks in when
:
fun main(){
var numSection = 4
when(numSection) {
1, 2, 3, 4 , 5 -> println("Primary Section.")
6, 7, 8, 9, 10 -> println("Secondary Section.")
11, 12 -> println("Higher Secondary Section.")
else -> println("College")
}
}
**Output: Primary Section**
In the above when
statement, we have combined 1 to 5 in one block. Similarly, 6 to 10, and 11 and 12. We don’t need to create each block separately.
Difference between when
in Kotlin vs. switch
in Java:
- In Kotlin, a
break
statement is not required, but in Java it is. when
sis used to check range data butswitch
is not.when
is more flexible thanswitch
.when
supports type check butswitch
does not.when
supports different types of data type check butswitch
does not.switch
has limitations, and only supports primitive types,enum
andstring
, butwhen
does not.- The syntax difference between
when
andswitch
is as follows:
// kotlin's when syntax
when( expression ){
condition ->
…………
condition ->
……………
else ->
……………
}
// Java's switch syntax
switch(……){
case :
break;
case :
break;
default :
break;
}
Using Kotlin when expression
Syntax of when
In Kotlin, when
is the replacement of multiple if... else
expressions. Next we’ll explain how to wrap multiple if... else
expressions into one when expression. The syntax of when is as follows:
// Syntax
when( expression ){
condition ->
…………
condition ->
……………….
else ->
……………..
}
// Example
fun main(){
val month = 4
val result = when (month) {
1 -> "Jan"
2 -> "Feb"
3 -> "Mar"
4 -> "April"
5 -> "May"
6 -> "June"
7 -> "July"
8 -> "Aug"
9 -> "Sept"
10 -> "Oct"
11 -> "Nov"
12 -> "Dec"
else -> "Invalid Month."
}
println("Month is $result")
}
**Output: Month is April**
Let us explain how it is executed, step-by-step:
- The when (month) is evaluated once
- The value of month variable compared with each branch
- Each branch starts with value followed by -> and result
- If any branch is matched, the corresponding block will be executed
- If no branch is matched, the else block will be executed
Compare when
to if... else
in Kotlin
The main difference between when and if...else
is code readability. when
statement has better control than if…else
statement. In Kotlin, when
, compile the code at once and execute it faster than the if... else
statement. If...else
statement executes each blog one by one, so it takes more time. Here’s an example of code readability:
fun main(){
var bookId = 2
if(bookId == 1){
println("Book price: $9.99")
}else if (bookId == 2){
println("Book price: $19.99")
}else if(bookId == 3){
println("Book price: $29.99")
}else{
println("Book ID does not match")
}
}
**Output: Book price: $19.99**
As you can see, the Kotlin code is lengthy and complex to learn. But, by using when
expressions, you can combine those codes. Here’s how it looks after combining:
fun main(){
var bookId = 2
when(bookId) {
1 -> println("Book price: $9.99")
2 -> println("Book price: $19.99")
3 -> println("Book price: $29.99")
else -> println("Book ID does not match")
}
}
**Output: Book price: $19.99**
Advanced Usage of when
There are several advantages to when
in Kotlin. It allows us to combine different cases in one by comma (,)
. Only one case must execute at a time. It means it performs as an OR
operator. Here’s an example to get started:
fun main(){
var numEven = 3
when(numEven) {
1 -> println("Even Number")
3 -> println("Even Number")
5 -> println("Even Number")
else -> println("Unknown Number")
}
}
**Output: Even Number**
We can see the first three cases are the same statement, so we can combine those into one by comma (,)
. Let’s see the result:
fun main(){
var numEven = 3
when(numEven) {
1,3,5 -> println("Even Number")
else -> println("Unknown Number")
}
}
**Output: Even Number**
Now if we pass numEven
1, 3 or 5, the first block will execute. There’s no need to define three cases separately.
Another advantage of when
is that it helps us check series of numbers in one line using an operator. Here’s an example:
fun main(){
var numEven = 101
when(numEven) {
0 -> println("Number is ZERO.")
in 1..100 -> println("Number between 1 and 100.")
else -> println("Number is greater than 100")
}
}
**Output:Number is greater than 100**
In this example, we have executed from 1 to 100 in single lines of code using an operator.
Using when with enum
An enum in Kotlin is a special kind of predefined data type that stores constant values. The keyword enum is used to declare in Kotlin. Here is an example of how we can use it through when:
enum class Color { RED, GREEN, YELLOW }
fun main(){
val signalColor: Color = Color.RED
val message = when (signalColor) {
Color.RED -> "STOP"
Color.GREEN -> "GO"
Color.YELLOW -> "SLOW"
}
println(message)
}
**Output: STOP**
Here we define an enum class called color, which has three values of red, green, and yellow. Depending on selecting the corresponding when cases will be executed.
Using when with lambda functions:
A lambda function is an anonymous function that is executed and returns only one expression. We can easily use the lambda function in when. Below, an example show some arithmetic operation through a lambda function within a branch:
enum class MathOperator { ADD, SUB, DIV }
fun main(){
val selectOPerator: MathOperator = MathOperator.SUB
when (selectOPerator) {
MathOperator.ADD -> {
val myLf: (Int) -> Unit= {result: Int -> println(result) }
addNumber(5,10,myLf)
}
MathOperator.SUB -> {
val myLf: (Int) -> Unit= {result: Int -> println(result) }
subNumber(15,10,myLf)
}
MathOperator.DIV -> {
val myLf: (Int) -> Unit= {result: Int -> println(result) }
divNumber(10,5,myLf)
}
}
}
fun addNumber(a: Int, b: Int, lam: (Int) -> Unit ){
val add = a + b
lam(add)
}
fun subNumber(a: Int, b: Int, lam: (Int) -> Unit ){
val sub = a - b
lam(sub)
}
fun divNumber(a: Int, b: Int, lam: (Int) -> Unit ){
val div = a/b
lam(div)
}
**Output: 5**
Smart casting with when
Smart casting in Kotlin is the most powerful feature. We used this expression for smart cast-in when. Using this technique, we can check any data type based on certain conditions. Here’s an example of how to check:
fun checkValue(value: Any) {
when (value) {
is String -> println("$value is a String")
is Int -> println("$value is an integer")
is Boolean -> println("$value is a Boolean")
else -> println("Unknown type")
}
}
fun main() {
checkValue("Hello")
checkValue(4)
checkValue(false)
checkValue(1.1)
}
**Output:
Hello is a String
4 is an integer
false is a Boolean
Unknown type**
Conclusion
With the when expression, we can easily combine lengthy sections of code into a small line. We can define several behaviors using when expressions, like smart casting, in operator, lambda function, enum, single block with comma (,), null check, and so on. There is no break keyword used.
That’s a great evolution offered by Kotlin programming language over the traditional Java switch statement, we are lucky to be Kotlin developers and be able to write Android applications using Kotlin language and take advantage of the evolution it provides over Java.
Expect The Unexpected!
Debug Faster With Bugfender