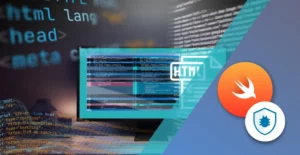
10 Minutes
Using Swift Vapor as a Backend Technology
Fix Bugs Faster! Log Collection Made Easy
Introduction to Swift as a backend technology
Swift is a powerful open source programming language created by Apple in 2014 for the iOS, iPadOS, macOS, watchOS, and tvOS, known for its modern syntax, safety features and fast performance.
Designed as a successor to Objective C, Swift has become a hugely popular choice for frontend and mobile app development, and it’s also shaping up to be an excellent choice for server-side development.
Table of Contents
Swift overview and evolution for backend development
Swift was designed to help new developers with its clean, concise syntax, while retaining benefits for power users.
The language provides modern, safe programming patterns, along with type safety, clean syntax and outstanding performance. What’s more, Swift was built as an open-source project, which has allowed the creation of frameworks such as as Vapor and Kitura. These frameworks are equipped with tools and libraries that enable us to write fully fledged server-side applications in Swift, handling HTTP requests, database integration and authentication management. This makes the language ideally suited to full stack development.
Four key reasons to use server side Swift for web development:
1) Performance: Swift performs strongly against C++, which is one of the fastest languages around, and it’s almost always faster than interpreted languages like Python and Ruby. Famous for its non-blocking I/O operations, Swift’s performance in CPU-bound tasks is at least the equal of its rivals, due to its compiled nature and efficient concurrency handling through the async/await syntax in Swift 5.5.
2. Safety and reliability: Swift forces the handling of errors, making code not only safer but easier to read and maintain later, reducing runtime crashes and issues in the production. The compiler makes sure to warn and show all errors throughout development.
3. Compatibility with Apple ecosystem: Developing a full-stack application in Swift enables us to share code, models, and business logic between frontend and backend components of an iOS, macOS, or server application. This allows for efficient and shorter software development lifecycles. And while Node.js, Ruby, and Python can serve as the backend solution for an app that has been developed in Swift, they don’t offer the compatibility advantages of using Swift at the server side.
4. Vibrant community and support: As a newcomer in backend development, Swift has quickly built a vibrant and highly supportive community. It is completely open-source, accepting contributions from developers all over the world, and enables a rich ecosystem to easily build apps with libraries, frameworks, and more. The frameworks Vapor and Kitura are supported by substantial documentation, a vast number of tutorials, and thriving community support, making it very easy for developers to choose Swift for their backend projects.
Swift server-side frameworks and tools
We’ve already introduced Vapor and Kitura. Now, let’s explore them in more detail.
Vapor Framework (https://vapor.codes/)
Vapor is a web framework that uses Swift as a server-side language, providing an expressive platform for implementing web applications and services. Vapor takes Swift’s strong typing and adds asynchronous features to provide a optimized, safe environment for server-side backend development.
Features of Vapor
i) Asynchronous programming model using Swift’s async/await makes it easy to write non-blocking Swift code.
ii) Fluent ORM is used to work with Swift databases, supporting MySQL, PostgreSQL, and SQLite.
iii) Vapor has powerful routing capabilities to handle HTTP requests.
iv) Vapor provides capabilities for Authentication & Authorization to secure the applications.
v) Vapor supports the WebSockets for real-time updates and communication.
Vapor is a great choice if high performance and scalability are among your key criteria, you intend to deploy your solutions on either Linux or macOS, and you are already handy with Swift. It’s suitable for everything from simple APIs to complex web applications and services.
Kitura Framework (https://www.kitura.dev/)
Kitura is an IBM lightweight, modular framework for developing server-side Swift applications. It is designed in a simple but powerful way, with tools that make it easy to rapidly and easily create web apps and RESTful APIs.
Features:
i) Kitura’s simplicity and speed are great for developers of all experience grades.
ii) The Model, View and Controller (MRC) pattern of Kitura is a variation of the traditional MVC pattern, made especially for server-side development.
iii) Kitura supports the Swagger developer portal for generation of API docs.
iv) Kitura supports CouchDB integration and Codable routing for handling JSON files.
v) It supports Prometheus, a powerful tool for monitoring.
Kitura is ideal for those who want simple but effective tools when developing RESTful APIs or web services, and is particularly great when integrating with IBM Cloud services or CouchDB.
Getting Started with Swift on the server
The following section will help you set up a working environment, create a simple REST API server, and introduce you to the most basic concepts of server-side programming in Swift.
Installation and setup
We need to install Swift on the development machine. Swift mainly runs on macOS, Linux, and Windows, but setting Swift up on these operating systems requires different steps. Installing Xcode from the Mac App Store includes the Swift compiler. Note that Swift can be installed through Xcode.
Choose a Framework
Technically it is possible to write server-side code using Swift without a framework, but both Vapor and Kitura are capable of doing things like processing HTTP requests, working with databases, and more backend tasks. In this case, we will use Vapor.
Install Vapor
To start your Backend using Vapor, you need to install it by running this command in your terminal (on macOS or Linux):
brew install vapor
This command will install Vapor on your computer.
Building a Simple REST API Server
Now let’s create a basic “Hello Bugfender” REST API using Vapor:
Create a New Vapor Project
Open your terminal and run this command:
vapor new HelloBugfender
This command creates a new directory called HelloBugfender
with a Vapor project template.
Navigate to project directory
Change into your created project’s directory:
cd HelloBugfender
Build the project
Now build the project to fetch its dependencies and compile it for the first time:
vapor build
Run the project
Start the server with the run
command:
vapor run
By default, the Vapor app runs on localhost:8080
.
Define a route
To define a route, open the Sources/App/routes.swift
file. Here, you are able to define a simple route that would return “Hello, Bugfender!” when accessed. Replace the contents of routes.swift
with this code:
import Vapor
func routes(_ app: Application) throws {
app.get { req in
return "Hello, Bugfender!"
}
}
Test the API
When the server is running, open a web browser and open http://localhost:8080/
. You should see “Hello, Bugfender!” displayed.
Key concepts in Swift for backend programming
The async/await-powered Swift concurrency model for server programs is able to handle I/O bound tasks well. Error handling
It’s best to keep things safe and predictable on your server with Swift. Learn to work with try
, catch
, and throw
to manage whatever server-side errors come your way.
Routing
Define routes to handle different HTTP requests, such as GET, POST, PUT, and DELETE.
Middleware
Middleware software, installed between the server and your application, can be used for logging, authentication and body parsing.
Models and databases
Server applications interact with databases, so you need to define the models and use ORMs such as Fluent in Vapor framework for database interactions.
Serialization and deserialization
Working with JSON data is one of the most common activities we carry out during backend development and integration. It doesn’t have to be complex in Swift. In fact, its Codable
protocol makes things simple.
Developing a real-world application
The development process with Swift on the server ranges from initial planning and design of the implementation through to security integration. It helps ensure delivery of robust, secure and scalable backend apps.
Planning and designing a Swift backend application
You need to first define the functionalities of application and the problem the app will solve. This will help you prioritize and focus on the most fundamental and essential features first. Then you need to choose the architecture, whether it is MVC, Model-View-View-Model (MVVM) or microservices architecture. Selecting the right architecture is essential and will enable us define the scalability of the app. Once you’ve done this, it’s time to focus on the data modelling, which refers to the entity and the relationship in the data. This forms the very basis of the project, giving us a clear understanding of how data will be saved, pulled, and manipulated in the application.
Now, we get to the cool stuff: the tech stack, frameworks, databases, and other tools you are going to use. For example, if the app is developed on the server side in Swift, you might use Vapor, a database like PostgreSQL, or other libraries for things like authentication and data validation.
Database integration: options and examples
Mainly Swift backend devs use SQL-based databases such as PostgreSQL or MySQL, although they may also consider NoSQL databases for use with Swift.
Of the SQL databases, one of the most popular choices is PostgreSQL because of its strength, features, and easy compatibility with ORM. Fluent is Vapor’s ORM to handle the database interactions the json way.
Here are some tips that should help you along the way:
- Include the Fluent PostgreSQL package in your
Package.swift
file. - With
configure.swift
, establish the connection of the PostgreSQL database to your real database URL, or to specific parameters like host, username and password. - Create the Swift models conjson formed to
Model
andContent
protocols and map them to your DB schema. - Use the Fluent migration tool to manage DB schema changes over time in the development.
Authentication and security practices
It’s important to choose the authentication method fit for your app. For web APIs, there are several popular options, such as JWT (JSON Web Token) and OAuth methods. JWT merely provides stateless authentication, whereas OAuth is bigger and has more flexibility, so you can use it for all third-party integrations.
How to implement JWT in-app
We need to use the library ajsonwebtoken
to issue and validate JWT tokens. Be sure to check issued tokens for every request with secure endpoint to ensure the request is authentic.
OAuth integration:
If your application needs OAuth integration, it would be better to consider using some existing library or service that Swift already supports. For those apps that need to be authenticated from outside services like Google and Facebook, this works perfectly.
Challenges and considerations
Swift for server-side development has its strengths and tradeoffs with traditional backend technologies. These challenges and considerations are important to keep in mind, as they will certainly impact you in your day-to-day work.
Let’s look at some of them now.
Comparison with traditional backend technologies
Swift offers high performance. In fact, it often outperforms other dynamically typed languages like Python and Ruby for intensive computation tasks. Swift should be selected according to the needs of the app in terms of performance.
Swift has taken care to be typesafe with its modern syntax, with a focus on security achieved through features such as optional types and error handling. Other languages, such as JavaScript (with Node.js) are very lenient and dynamic, which can provide a double-edged sword: if not properly handled and implemented, they can provoke an increase in bugs.
However, other languages like Java, C#, and PHP present a huge ecosystem of libraries and frameworks for all pieces of functionality (which is no surprise, given they’ve had 30 years to develop them). Swift’s server side ecosystem, in contrast, is still growing and young.
What’s more, deployment of Swift is more complex than that of other languages, and while Swift has a very active and growing community, the server side has a smaller community than traditional backend languages.
To sum up
Swift has now become a big choice for server-side programming in terms of flexible performance and community dedication. In this article we have walked through several good aspects related to performance benefits, safety features, and community support. We have also described starting with Swift on the server, introduced frameworks like Vapor and Kitura, discussed the deployment and scaling of Swift backend services, and loads of other cool stuff.
If you want to move forward with backend development using Swift, we recommend you to read our next article full of tips on how to write backend code in Swift.
We hope you enjoy using Swift in your backend development, and if you have any questions, don’t hesitate to reach out. Happy coding!
Expect The Unexpected!
Debug Faster With Bugfender